What is the meaning of "return" in Java programming language?
The return keyword in Java is arguably the least utilised. Almost all programming languages provide this feature, which enables the programmer to select the return result of the current method.
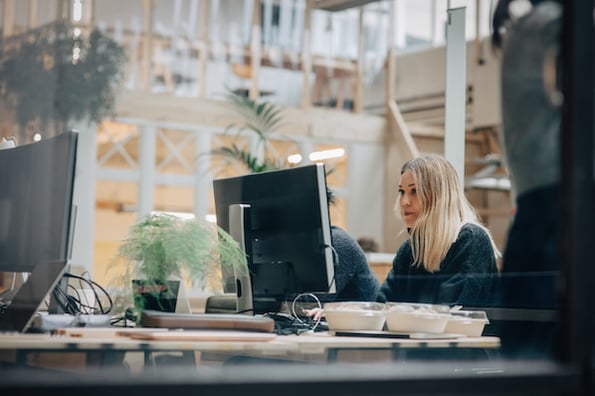
In this post, we’ll cover the various use cases of the return keyword, along with examples, and help you take advantage of this feature.
What is the Java return keyword?
The return keyword in Java causes a method to return a value. When the keyword is detected, the method will immediately return the value. This indicates that the method will end after the return keyword and that any local variables it has established will be deleted.
The return keyword can be used by programmers with or without a value. A value will be returned to the caller if one is provided. A null value will be returned in all other cases.
When utilising the return keyword, there are a few recommended practises to follow. Let's examine how this keyword operates in functions and the kinds of data it might return.
How to Use return in Java
Return statements redirect the programme outside of the execution of that block, similar to how a break statement does in a for loop. In other words, a return statement hands back control to the caller immediately after exiting the current method.
This is similar to how a break statement immediately exits a for loop and transfers control back to the enclosing code block. To understand how this works, consider the following example:
If you call the function in the code above with the number 8, it will return the string "Number less than 10" and go on to the caller. The function also returns "Number more than 10 but less than 100" if parameter an is less than 100. The programme multiplies a by 10 and outputs "Number larger than or equal to 100" if the parameter is more than or equal to 100.
A return type must be specified in the definition of every Java method. Use void as the return type if there is nothing to be returned. There are various return kinds that can be divided into two categories. Non-primitive types can represent things like classes, arrays, and doubles, while primitive types like int, float, and double represent raw values.
We called the method function before ("Hello World"). When called with a string as an input, the display() function returns an instance of the DisplayClass object. This demonstrates that we can also return non-primitive types from a method, such as a class or interface.
A function must only return data of the type for which it was declared to return data. A build time error will be encountered otherwise. This is due to the fact that a method's return type is a component of the method's signature, which must coincide with the signature of the variable used to hold the method's return value.
You cannot return a string, for instance, if the method's return type is an integer.
Additionally, the parameters must be passed to the method in the same order as they are accepted by it. This is so that the method can only access the parameters in the order that they are passed to it. The method won't be able to use the parameters correctly if the parameters are not given in the right order.
Consider the example below:
Now, if you don’t pass a and b in the right sequence, you might run into unexpected output.
Furthermore, remember to use the void type when your function doesn’t return a value. Consider the following example, which has a function with an int argument that you’re printing:
Demonstration with Simple Arithmetic
Consider a function that takes two integer arguments and returns the largest of the two, as shown below:
Similarly, you can perform some arithmetic operations on integers to obtain the appropriate results. For example:
Java return Best Practices
There are a few best practises to remember while using the Java return keyword.
First, wherever possible, return a value from a method. If there is nothing to return, void is also returned. This will make it easier to ensure that your code is dependable and troubleshootable.
Make sure a method returns the appropriate data type next. Make sure your method delivers an integer value if a method expects an integer return type.
Last but not least, it's always a good idea to explain what a method returns so that other developers can comprehend your code with ease.
This final example shows all of these best practices in one simple function — can you identify them all?
When utilising the return keyword, make sure to adhere to these straightforward recommended practises to keep your codebase dependable and simple to maintain.
return Learn the fundamentals of Java.
A function's execution is halted and the intended result is returned with the return keyword. It will use void as a return type even if there is nothing to return. In addition, you can halt the execution of a function using return without a value.
Remember that the return type of the method and the type of value it returns must match the type of the variable where the returned value is stored when implementing return statements.
Comments
Post a Comment